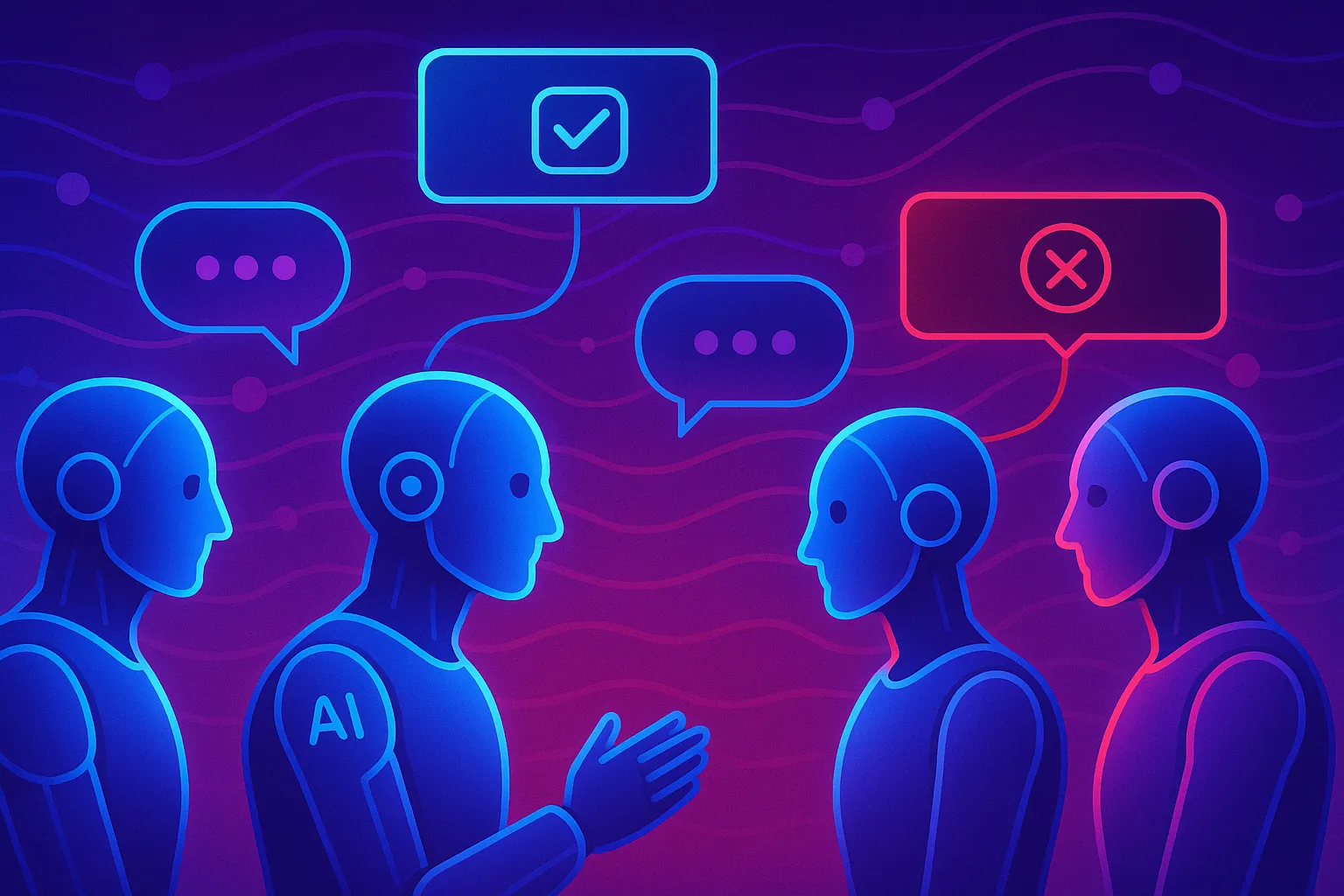
Understanding Selection and Termination Strategy functions in .NET Semantic Kernel Agent Framework
Explore how Selection and Termination Strategy Functions in the Semantic Kernel Agent Framework for .NET manage multi-agent conversations by choosing the next speaker and deciding when to end the chat.
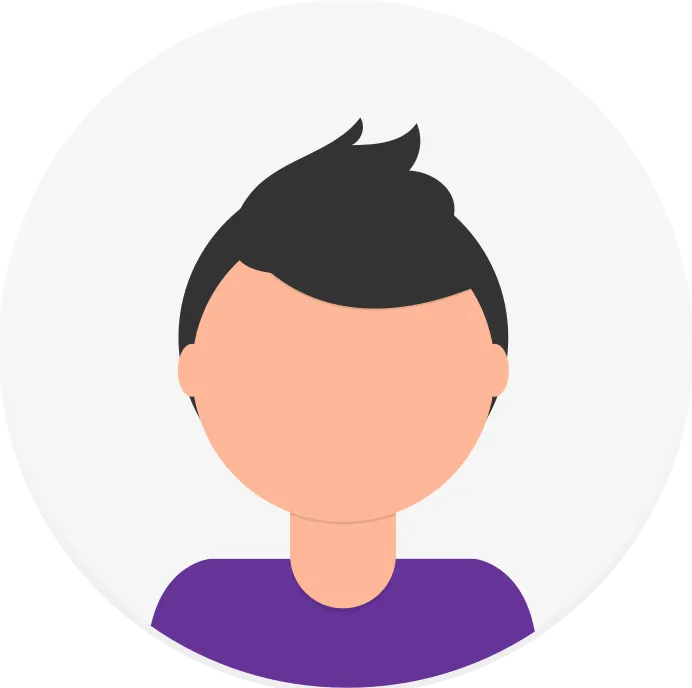
The Microsoft Semantic Kernel Agent Framework provides powerful tools for building sophisticated AI agent systems that can work together to solve complex problems. One of the most exciting capabilities is creating multi-agent conversations where AI agents can collaborate, discuss, and reach conclusions autonomously. This blog explores the critical components that control these interactions: Selection and Termination Strategy Functions.
Selection Strategy Function determines which agent speaks next in a conversation, while Termination Strategy Function decide when a conversation should end. Together, they create structured, productive agent interactions that avoid common pitfalls like endless loops or inefficient exchanges. Understanding how to implement and customize these strategies will help you build more effective AI agent systems.
Learn about:
- Building AI Agent using Semantic Kernel Agent Framework
- Building Multi‑Agent AI Workflows with Semantic Kernel Agent Framework in .NET
AI Agent Group Chat and Strategy Functions
In multi-agent systems, several AI agents with different roles, expertise, or personalities work together to address user queries or solve problems. For example, you might have a developer agent that writes code, a critic agent that reviews it, and a documentation agent that explains it. The Semantic Kernel framework provides built-in mechanisms to orchestrate these multi-agent conversations through:
Selection Strategy: KernelFunctionSelectionStrategy
determines which agent among a group should respond next in a conversation. It evaluates the current conversation context and selects the most appropriate agent to continue the dialogue based on specific criteria.
// Basic instantiation of a KernelFunctionSelectionStrategy
var selectionStrategy = new KernelFunctionSelectionStrategy(
function: selectionFunction,
kernel: kernel,
InitialAgent = agentName, // Always start with this agent.
AgentsVariableName = "agents",
HistoryVariableName = "history", // The prompt variable name for the history argument.
ResultParser = result => result.GetValue<string>() ?? "DefaultAgent", // Returns the entire result value as a string.
EvaluateNameOnly = true, // Only include the agent names and not the message content
);
Key Properties
- Function: The KernelFunction contains the prompt template that rules “who speaks next” based on history.
- InitialAgent: An optional fallback agent if no selection is made in the first turn.
- HistoryVariableName: The name of the kernel argument (e.g., “history”) carrying the chat history into the function.
- HistoryReducer: An IChatHistoryReducer to truncate history (e.g., keep the last 10 interactions) for prompt size control.
- AgentsVariableName: The argument key for the list of agent names available for selection.
- EvaluateNameOnly: When true, only agent names (not full message content) are passed to the function, reducing context size.
- ResultParser: A callback function that converts the function result into agent selection criteria.
- UseInitialAgentAsFallback: If selection fails, controls whether to default back to InitialAgent
Termination Strategy: KernelFunctionTerminationStrategy
signals when a chat should end by evaluating a KernelFunction against the history (and optionally other data). Without these strategies, agents could get stuck in endless loops or inefficient back-and-forth exchanges. Proper implementation ensures conversations flow naturally and reach meaningful conclusions.
// Basic instantiation of a KernelFunctionTerminationStrategy
var terminationStrategy = new KernelFunctionTerminationStrategy(
agents: new[] { agentName }, // Agents that can trigger termination
function: terminationFunction,
kernel: kernel,
resultParser: result => "TERMINATE" == result.GetValue<string>(),
maximumIterations: 10, // Safety limit
// Save tokens by not including the entire history in the prompt
HistoryReducer = new ChatHistoryTruncationReducer(5)
);
Key Properties
- Function: The KernelFunction prompt asks “Are we done?” (e.g., “If done, respond with ‘done’”)
- Agents: Specifies which agents’ outputs to consider for termination.
- AgentVariableName: The kernel argument key for the agent name passed to the function.
- HistoryVariableName: The name of the argument holding chat history (default is “history”)
- HistoryReducer: Trims the history to the last n messages to control prompt length (e.g., last 1 for succinctness)
- MaximumIterations: A hard cap on turns (e.g., 10) after which the chat ends regardless of function output
- ResultParser: A callback that inspects the LLM’s function result (e.g., checking if the result contains “done” case‑insensitively) to set IsComplete
Implementation of Selection/Termination Strategy Functions:
We will continue with the example from this Building Multi‑Agent AI Workflows with Semantic Kernel Agent Framework in .NET Blog.
1. Create Kernel Function Prompt:
// Define a selection function that alternates between drafter and approver
KernelFunction emailSelectionFunction = AgentGroupChat.CreatePromptFunctionForStrategy(
"""
Determine which agent should take the next turn based on the last speaker.
- After EmailDrafterAgent, it's EmailApproverAgent's turn.
- After EmailApproverAgent, it's EmailDrafterAgent's turn.
State only the agent name to go next (no extra text).
Candidates:
- EmailDrafterAgent
- EmailApproverAgent
History:
{{$history}}
""",
safeParameterNames: "history"
);
KernelFunction emailTerminationFunction = AgentGroupChat.CreatePromptFunctionForStrategy(
"""
Check whether the most recent emailApproverAgent response contains an approval indicator.
If the response contains the word "APPROVED" (case‐insensitive), reply with exactly "yes".
History:
{{$history}}
""",
safeParameterNames: "history"
);
2. Configure the group chat to use these strategies:
var chat = new AgentGroupChat(emailDrafter, emailApprover)
{
ExecutionSettings = new()
{
// Terminate when approver says "yes"
TerminationStrategy = new KernelFunctionTerminationStrategy(emailTerminationFunction, _kernel)
{
Agents = new[] { emailApprover },
HistoryVariableName = "history",
ResultParser = result =>
{
var val = result.GetValue<string>() ?? string.Empty;
return val.Contains("yes", StringComparison.OrdinalIgnoreCase);
},
MaximumIterations = 10
},
// Alternate turns between drafter and approver
SelectionStrategy = new KernelFunctionSelectionStrategy(emailSelectionFunction, _kernel)
{
InitialAgent = emailDrafter,
HistoryVariableName = "history",
//use the raw function output as the next agent name
ResultParser = result => result.GetValue<string>() ?? EmailDrafterName,
HistoryReducer = new ChatHistoryTruncationReducer(1)
}
}
};
- The selection function alternates between drafter and approver by reading the last speaker from history and returning the next.
- The termination function checks for an approval keyword (yes) in the approver’s output to end the chat.
Output
-
Turn 1: EmailDrafterAgent drafts an email based on the user prompt.
-
Turn 2: SelectionStrategy invokes the selection prompt, sees EmailDrafterAgent spoke last, and selects EmailApproverAgent.
-
Turn 2: EmailApproverAgent reviews the draft and responds.
-
Termination Check: TerminationStrategy runs the termination prompt; if the approver’s text contains “yes”, the conversation stops.
-
Repeat: If not approved, the loop continues, alternating agents until approval or 10 turns elapse, at which point the chat ends automatically.
Common Challenges and Solutions
Selection Strategy Issues
Problem: Selection logic chooses the wrong agent for a given context.
Solution: Refine your prompting in the selection function to better analyze the conversation semantics.
Problem: Agent selection gets stuck in a ping-pong pattern between two agents.
Solution: Add logic to your selection strategy that detects and breaks repetitive patterns.
Termination Strategy Issues
Problem: Conversation terminates too early before reaching a satisfactory conclusion.
Solution: Make your termination criteria more specific and increase the required confidence threshold.
Problem: Termination doesn’t trigger despite the presence of termination keywords.
Solution: Ensure your result parser correctly handles case sensitivity and string formatting.
Conclusion
Selection and Termination Strategy Functions are essential components for building effective multi-agent systems with Microsoft’s Semantic Kernel Agent Framework. They provide the orchestration layer that ensures conversations flow logically between the right agents and reach appropriate conclusions.
By understanding and customizing these strategies, you can create sophisticated agent interactions that solve complex problems while avoiding common pitfalls like circular discussions or premature termination.