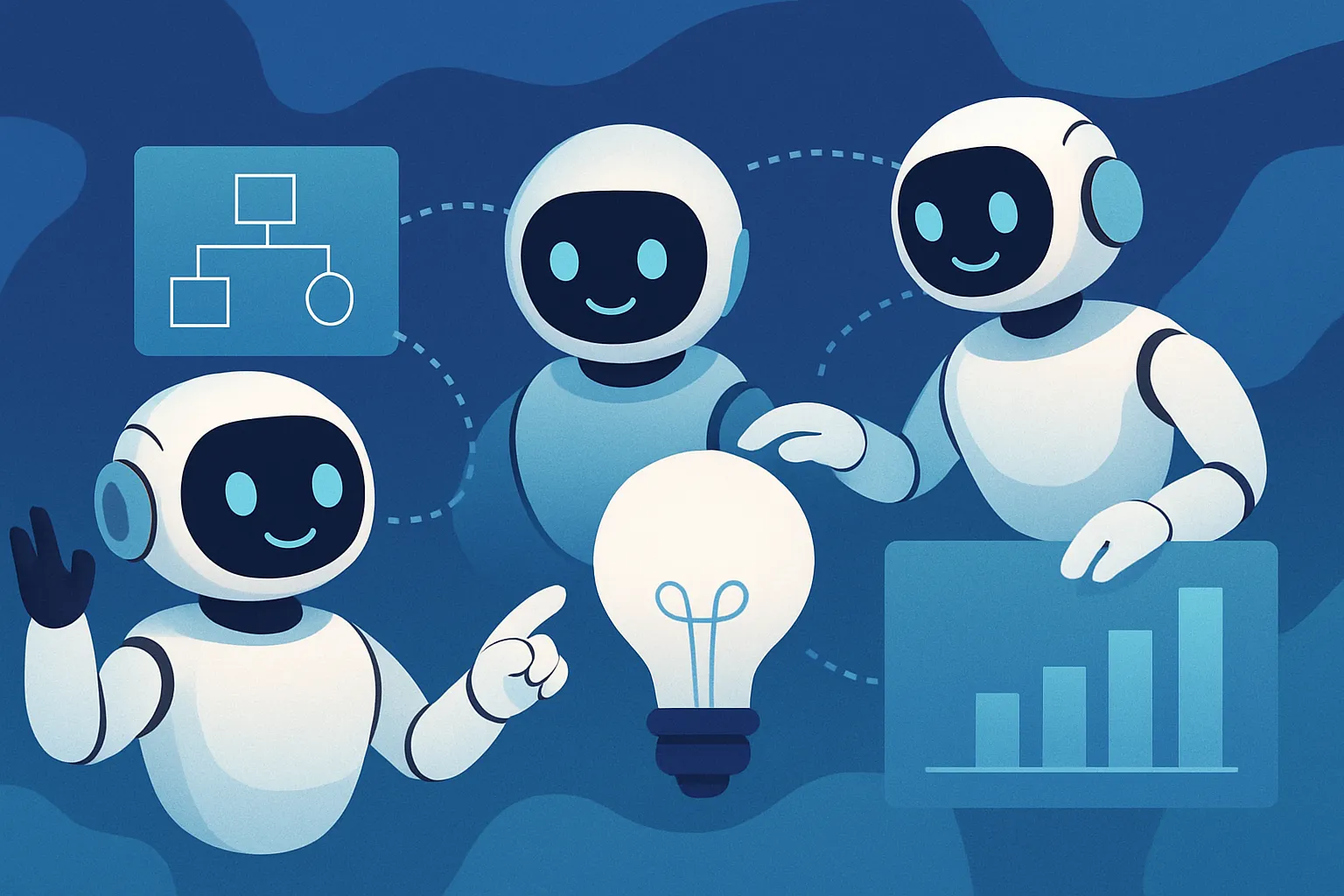
Building Multi‑Agent AI Workflows with Semantic Kernel Agent Framework in .NET
Explore how multi-agent systems enable AI agents to collaborate seamlessly, enhancing efficiency and scalability. Learn to implement this approach using Microsoft's Semantic Kernel, orchestrating specialized agents to automate tasks like email drafting and approval.
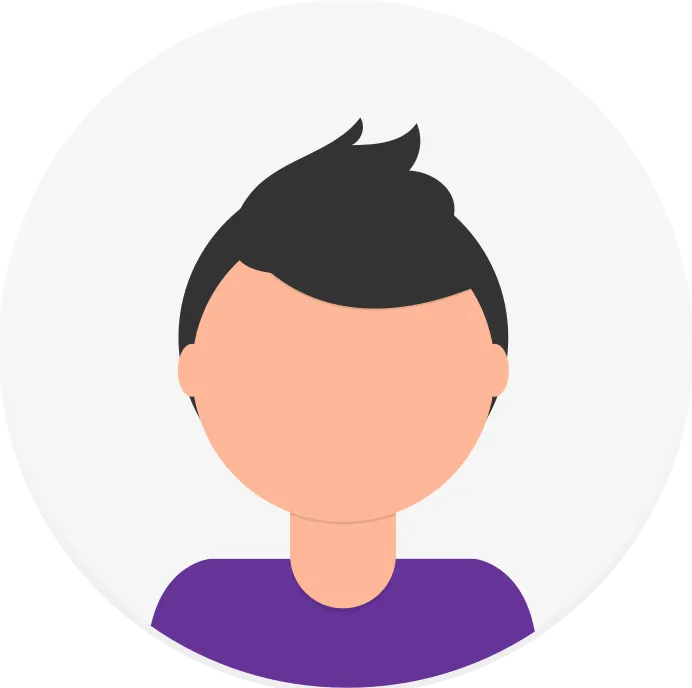
What is a Multi-Agent System?
A multi-agent system consists of multiple AI agents that work together to address intricate tasks by breaking them down into smaller, more manageable subtasks. In contrast to single-agent architectures, which depend on one AI entity to handle every aspect of a task, multi-agent systems allocate responsibilities among specialized agents, each concentrating on a particular function or area of expertise.
Key Concepts in Agent Framework
To effectively build multi-agent systems, you need to understand several key components that enable agent interactions and workflows.
- Agent Chat: Agent Chat is the basic communication channel where an individual agent interacts with users. In Semantic Kernel, this is handled through the
ChatCompletionAgent
class. - Agent Group Chat: AgentGroupChat allows multiple agents to talk to each other in a structured way. This coordinated chat helps agents collaborate more effectively.
- Agent Selection: Agent Selection means choosing the right agent to handle a specific part of a task. This can be rule-based (using set logic) or dynamic (based on the situation). Good selection makes sure each task is handled by the most suitable expert.
- Agent Termination: Agent termination refers to determining when agents should stop when their work is done to save resources. This might happen when a task is complete, a condition is met (like getting approval), or after a set number of steps.
- Agent Threads: Agent Threads keep the conversation history so agents don’t lose context. They help agents follow long interactions, remember past messages, and handle tasks in order or in parallel when needed.
Learn about Building AI Agent using Semantic Kernel Agent Framework.
Step-by-Step Implementation of Multi-Agent Systems with Semantic Kernel
This practical demonstrates a email drafting and approval workflow with two specialized agents.
1. Setting Up the Environment
The implementation begins with initializing Semantic Kernel and configuring the Azure OpenAI service. Configure the Kernel builder by loading AzureOpenAISettings and adding the Azure OpenAI chat completion service via AddAzureOpenAIChatCompletion(...)
public MultiAgentChat()
{
_config = new ConfigurationBuilder().AddJsonFile(configPath).Build();
var deploymentName = _config.GetSection("AzureOpenAISettings:Model").Value;
var endpoint = _config.GetSection("AzureOpenAISettings:Endpoint").Value;
var apiKey = _config.GetSection("AzureOpenAISettings:ApiKey").Value;
var builder = Kernel.CreateBuilder();
builder.AddAzureOpenAIChatCompletion(
deploymentName: deploymentName,
endpoint: endpoint,
apiKey: apiKey);
_kernel = builder.Build();
}
2. Defining Specialized Agents
To define the specialized agents, two ChatCompletionAgent
instances are created one for drafting and one for approving emails. Each agent is given clear instructions outlining its role, a unique name for identification during conversations, and access to the Semantic Kernel to leverage AI capabilities. The EmailDrafter
focuses on writing professional emails, while the EmailApprover
is responsible for reviewing drafts and offering feedback.
private const string EmailDrafterName = "EmailDrafterAgent";
private const string EmailDrafterInstructions = """
You are a corporate communications expert specializing in email composition.
Your responsibilities:
- Draft clear, professional emails based on brief descriptions
- Maintain appropriate tone for the recipient
- Ensure proper formatting and structure
- Revise based on feedback
""";
private const string EmailApproverName = "EmailApproverAgent";
private const string EmailApproverInstructions ="""
You are a communications manager reviewing outgoing emails.
Your role:
- Verify message clarity and professionalism
- Check for proper formatting and grammar
- Ensure compliance with company style guide
- Respond with either "APPROVED" or specific revision requests
""";
ChatCompletionAgent emailDrafter = new()
{
Instructions = EmailDrafterInstructions,
Name = EmailDrafterName,
Kernel = _kernel,
};
ChatCompletionAgent emailApprover = new()
{
Instructions = EmailApproverInstructions,
Name = EmailApproverName,
Kernel = _kernel,
};
3. Creating an Agent Group Chat
To enable collaboration between the agents, the code sets up an AgentGroupChat
that includes both the drafter and approver agents. It uses ExecutionSettings
to control the flow of the conversation, specifying how the agents will interact and when the chat should end. A custom EmailApprovalTerminationStrategy
is implemented to stop the chat once the approval criteria are met. Additionally, a maximum iteration limit is set to avoid infinite loops and ensure the process completes efficiently.
AgentGroupChat chat = new(emailDrafter, emailApprover)
{
ExecutionSettings = new()
{
TerminationStrategy = new EmailApprovalTerminationStrategy()
{
Agents = new[] { emailApprover },
MaximumIterations = 10
}
}
};
4. Implementing Custom Termination Strategy
The custom termination strategy ensures the conversation ends at the right moment. When the EmailApproverAgent
agent explicitly says “APPROVED” or after a predefined number of messages. This provides a clear and reliable endpoint for the workflow, preventing unnecessary loop once the objective is achieved.
private sealed class EmailApprovalTerminationStrategy : TerminationStrategy
{
// Terminate when the final message contains the term "APPROVED"
protected override Task<bool> ShouldAgentTerminateAsync(Agent agent, IReadOnlyList<ChatMessageContent> history, CancellationToken cancellationToken)
=> Task.FromResult(history[history.Count - 1].Content?.Contains("APPROVED", StringComparison.OrdinalIgnoreCase) ?? false);
}
4. Initiating the Multi-Agent Workflow
To start the multi-agent workflow, a message from the user is added, and InvokeAsync()
is called to let the agents respond. The system handles each reply step by step, allowing the agents to work together in a smooth and natural way. This continues until the conversation ends based on the termination strategy.
ChatMessageContent input = new(AuthorRole.User, "Write a chill email to the exec team about missing Q3 targets. Keep it lighthearted. Dont maintain any professionalism use slang words");
chat.AddChatMessage(input);
try
{
Console.WriteLine("Chat Progress:");
await foreach (ChatMessageContent response in chat.InvokeAsync())
{
Console.ForegroundColor = ConsoleColor.DarkGreen;
Console.WriteLine($"Agent:{response.AuthorName} ({response.Role}):");
Console.ResetColor();
Console.WriteLine(response.Content + "\n");
}
}
catch (Exception ex)
{
Console.WriteLine($"Chat failed: {ex.Message}");
}
Output
The output shows a conversation between two AI agents: EmailDrafterAgent and EmailApproverAgent. The EmailDrafterAgent drafts a casual email about missing Q3 targets. The EmailApproverAgent rejects it, pointing out that it’s too informal and suggests improvements like using a professional tone and clearer structure. The EmailDrafterAgent then rewrites the email with a respectful, constructive tone. This revised version is approved by the EmailApproverAgent, and the conversation ends successfully based on the defined termination strategy. This demonstrates how multiple agents can collaborate, review, and refine content to meet specific goals just like in a real-world workflow.
Conclusion
Multi-agent systems represent a significant advancement in artificial intelligence, enabling specialized agents to collaborate, adapt, and solve complex problems more effectively than single-agent models. By leveraging frameworks like Microsoft’s Semantic Kernel, developers can implement structured workflows that enhance efficiency, scalability, and resilience.