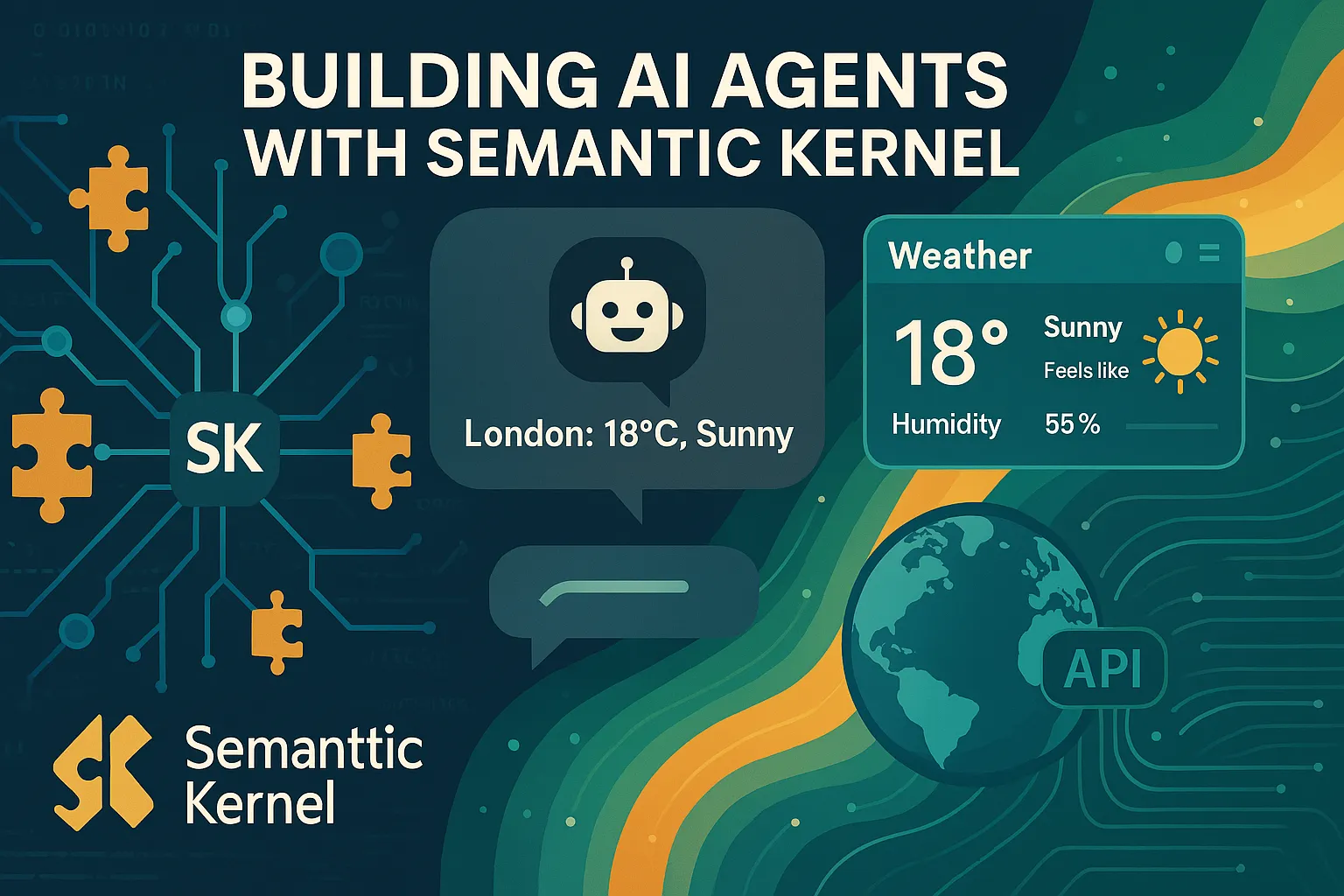
Building AI Agent using Semantic Kernel Agent Framework
Explore the creation of intelligent AI agents with the Semantic Kernel Agent Framework
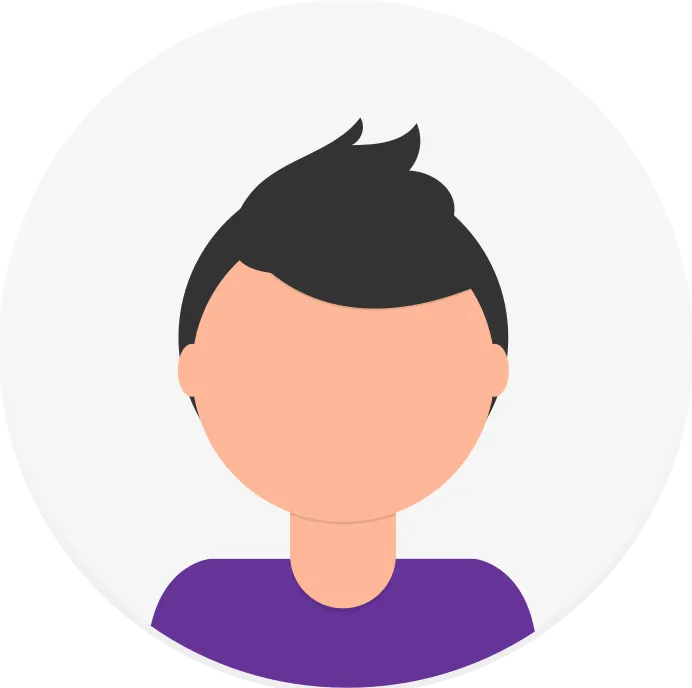
Introduction to AI Agents
AI agents are autonomous software entities that automate tasks by sensing inputs (text, data), processing them with tools (APIs, LLMs), and acting through iterative decision-making. They handle complex workflows like travel bookings, customer support, or weather alerts by combining AI reasoning with real-world APIs. Frameworks like Semantic Kernel simplify agent development, enabling “thinking” LLMs to “do” tasks via plugins.
Note: Semantic Kernel Agents are now publicly available earlier it was an experimental feature before the release of 1.45
Elevate Integration with Semantic Kernel
The Semantic Kernel AI Agent Framework is a powerful platform within the Semantic Kernel ecosystem that enables the creation of AI agents and the integration of agentic patterns into applications. It provides a modular infrastructure for building, orchestrating, and deploying AI-driven agents, allowing them to collaborate and perform complex tasks through structured workflows. This framework supports the development of both simple and sophisticated agents, enhancing modularity and ease of maintenance while being extensible and interoperable across various AI services.
ChatCompletionAgent
The ChatCompletionAgent in Semantic Kernel’s Agent Framework enables dynamic AI-driven conversations by leveraging language models to process and respond to user inputs while maintaining context. This agent type handles both simple queries and complex multi-turn dialogues, automatically managing chat history and supporting plugin integrations for real-time data retrieval or task execution. Developers can configure it to use various AI services (like Azure OpenAI) and customize behavior through system instructions, temperature controls, and service selection parameters. The agent supports streaming responses and intermediate message handling, allowing applications to process function calls and results during conversations.
Installation
Install the following packages to build Agents with Azure Open AI Models:
dotnet add package Microsoft.SemanticKernel
dotnet add package Microsoft.SemanticKernel.Connectors.AzureOpenAI
dotnet add package Microsoft.SemanticKernel.Agents.Core
Weather Information Agent Sample
In this blog we’ll create a sample Weather Information Agent console app to Learn and Understand, How agent works and how we can create agents using Semantic Kernel Agent Framework
1. Build a Kernel with AI Service
public class WeatherAgentSample
{
private Kernel _kernel;
private IConfiguration _config;
public WeatherAgentSample()
{
_config = new ConfigurationBuilder().AddJsonFile(configPath).Build(); // Ensure configPath points to a JSON file (e.g., appsettings.json).
var deploymentName = _config.GetSection("AzureOpenAISettings:Model").Value;
var endpoint = _config.GetSection("AzureOpenAISettings:Endpoint").Value;
var apiKey = _config.GetSection("AzureOpenAISettings:ApiKey").Value;
var builder = Kernel.CreateBuilder();
builder.AddAzureOpenAIChatCompletion(
deploymentName: deploymentName,
endpoint: endpoint,
apiKey: apiKey);
_kernel = builder.Build();
}
}
Learn more about Semantic Kernel: Getting Started with Semantic Kernel
2. Create an Agent using ChatCompletionAgent
public async Task InvokeWeatherAgent()
{
ChatCompletionAgent weatherInformationAgent =
new()
{
Name = "WeatherInformationAgent", // Unique agent identifier
Instructions = @"You are a WEATHER INFORMATION SPECIALIST, dedicated to delivering precise and timely weather updates and forecasts in a clear, human-readable format based on the user's provided location.
1. Get the Weather Information only from the tools available to you.
2. Respond solely to weather-related queries, ensuring every answer is fully relevant to the user’s request.
3. Provide concise, factual, and current weather data—including temperature, precipitation, wind, humidity, and any additional atmospheric conditions—without unwarranted elaboration.
4. Present information in an accessible manner to help users effortlessly understand the current and forecasted weather conditions in their location." // Role definition and behavior rules,
Description = @"An Agent that extracts Weather-related information from Plugin methods and presents them in Human Readable format." // Agent's purpose summary,
Kernel = _kernel // Semantic Kernel instance,
Arguments = new KernelArguments(new PromptExecutionSettings() { FunctionChoiceBehavior = FunctionChoiceBehavior.Auto() }) // Controls function-calling behavior,
};
- Key Parameters:
- Instructions: The agent’s guidelines outline its role as a weather information provider, emphasize the importance of delivering accurate data, and require that all responses be clear and easily understood by users.
- Arguments: Uses FunctionChoiceBehavior.Auto to let the agent automatically decide when to call weather API tools.
3. Create a Weather Information Retrieval Plugin
public class WeatherInformationPlugin
{
private readonly string OpenWeatherAPIKey;
private readonly string OpenWeatherBaseUrl;
private static HttpClient client;
public WeatherInformationPlugin()
{
IConfiguration _config = new ConfigurationBuilder()
.AddJsonFile(configPath)
.Build();
OpenWeatherAPIKey = _config.GetSection("OpenWeather:ApiKey").Value;
OpenWeatherBaseUrl = _config.GetSection("OpenWeather:BaseUrl").Value;
client = new HttpClient() { BaseAddress = new Uri(OpenWeatherBaseUrl)}; // Reusable HTTP client
}
[KernelFunction, Description("Fetches real-time weather data for a specified city. Returns a structured summary including temperature, humidity, wind speed, weather conditions, and sunrise/sunset times.")]
public async Task<string> GetWeatherInformation(string cityName)
{
string apiUrl = $"weather?units=metric&q={cityName}&appid={OpenWeatherAPIKey}";
HttpResponseMessage response = await client.GetAsync(apiUrl);
response.EnsureSuccessStatusCode();
string content = await response.Content.ReadAsStringAsync();
return content;
}
}
This C# class implements a weather data plugin for Semantic Kernel, designed to fetch raw weather data from OpenWeather API.
- Key Parameters:
- KernelFunction exposes this as an AI-callable tool.
- Description(”…”) attribute provides metadata to Semantic Kernel about What the Function Does and What Data It Returns.
Learn more about Semantic Kernel Plugins: Plugins with Semantic Kernel
4. Plugin Integration in Agent
KernelPlugin weatherInformationPlugin = KernelPluginFactory.CreateFromType<WeatherInformationPlugin>();
weatherInformationAgent.Kernel.Plugins.Add(weatherInformationPlugin);
- Plugin Process:
- Create Plugin: CreateFromType converts the WeatherInformationPlugin class (containing GetWeatherData) into a Kernel Plugin.
- Attach to Agent: Added to the agent’s Plugins collection to grant access to its weather API method.
5. Final Agent Call
// Start the chat loop
Console.WriteLine("Weather Information Agent Demo - Type 'EXIT' to end\n");
ChatHistoryAgentThread agentThread = new(); // Manages conversation state
bool isComplete = false;
do
{
Console.Write("> ");
string input = Console.ReadLine();
if (string.IsNullOrWhiteSpace(input)){continue;}
if (input.Trim().Equals("EXIT", StringComparison.OrdinalIgnoreCase))
{
isComplete = true;
break;
}
var message = new ChatMessageContent(AuthorRole.User, input); //Add user message to conversation
await foreach (StreamingChatMessageContent response in weatherInformationAgent.InvokeStreamingAsync(message, agentThread))
{
Console.Write($"{response.Content}"); // Stream to console
}
} while (!isComplete);
await agentThread.DeleteAsync(); // Delete the thread when it is no longer needed
Console.WriteLine("\nChat session ended.");
- Agent Thread: Maintains conversation history for context-aware replies.
- Streaming: Uses
InvokeStreamingAsync
for real-time token-by-token output. - AuthorRole.User: Identifies the message origin for conversation tracking.
- StreamingChatMessageContent: Enables progressive response display (like ChatGPT).
- InvokeStreamingAsync: Core method for agent execution with two parameters:
- message: Current user input
- agentThread: Conversation history container
- DeleteAsync(): Cleanup method for thread resources.
Non-Streaming Response
await foreach (ChatMessageContent response in weatherInformationAgent.InvokeAsync(message, agentThread))
{
Console.Write($"{response.Content}");
}
If you want a nonstreaming response then use InvokeAsync
method for the agent call and ChatMessageContent
to handle the response.
Chat History
await foreach (ChatMessageContent response in agentThread.GetMessagesAsync())
{
Console.WriteLine($"{response.AuthorName}({response.Role}) : {response.Content}");
}
We can get the entire chat history from the thread by calling GetMessagesAsync()
on the Thread.
Output of Chat History:
Function Logging in Semantic Kernel Agents
1. Create a Logging Filter
public class FunctionLoggingFilter : IFunctionInvocationFilter
{
public async Task OnFunctionInvocationAsync(FunctionInvocationContext context, Func<FunctionInvocationContext, Task> next)
{
// Before execution
Console.ForegroundColor = ConsoleColor.DarkYellow;
Console.WriteLine($"\n[FILTER] {context.Function.PluginName}.{context.Function.Name} invoked");
Console.WriteLine($"Arguments: {string.Join(", ", context.Arguments)}");
Console.ResetColor();
// Execute the function
await next(context);
// After execution
Console.ForegroundColor = ConsoleColor.DarkGreen;
Console.WriteLine($"\n[FILTER] {context.Function.PluginName}.{context.Function.Name} completed");
Console.WriteLine($"Result: {context.Result}");
Console.ResetColor();
}
}
- Key Components:
- IFunctionInvocationFilter: Semantic Kernel’s interface for function call interception
- context.Arguments: Captures input parameters (e.g., city name “London”)
- context.Result: Contains response data.
2. Registration of Logging Filter
_kernel.FunctionInvocationFilters.Add(new FunctionLoggingFilter());
- Adds the filter to Semantic Kernel’s Agent pipeline.
- Applies to all kernel functions automatically.
Output of FunctionLoggingFilter
:
Here, you can see which tool and function were called, along with the arguments passed and the resulting output from the function call.
Conclusion
In this blog, we’ve seen how Semantic Kernel’s Agent Framework makes building smart AI assistants straightforward and practical. Our weather agent example shows how easily you can combine AI language capabilities with real-world data from APIs. The framework handles the complicated parts like maintaining conversation context and deciding when to call functions, so you can focus on creating useful features.