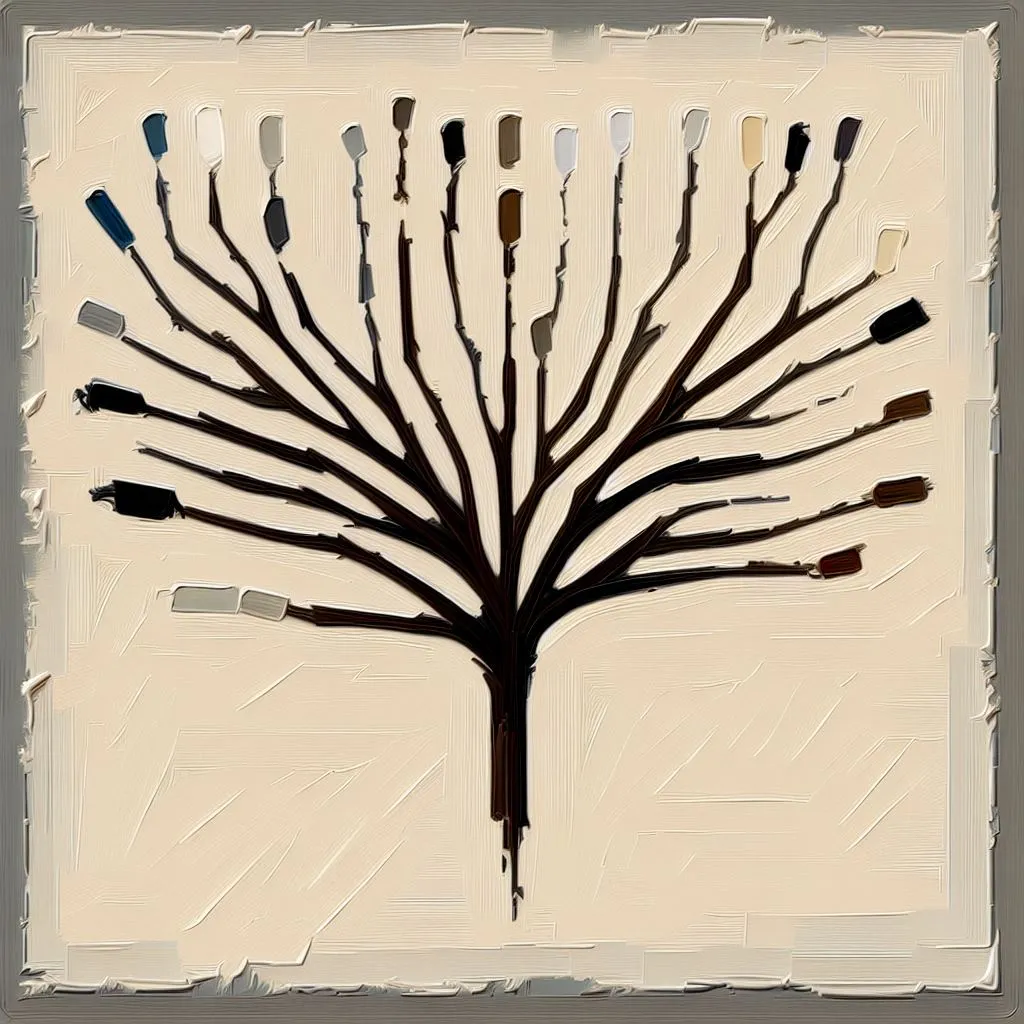
Setting up the Azure OpenAI API versions in Semantic Kernel
Managing Azure OpenAI API versions in Semantic Kernel, including both new and legacy approaches.
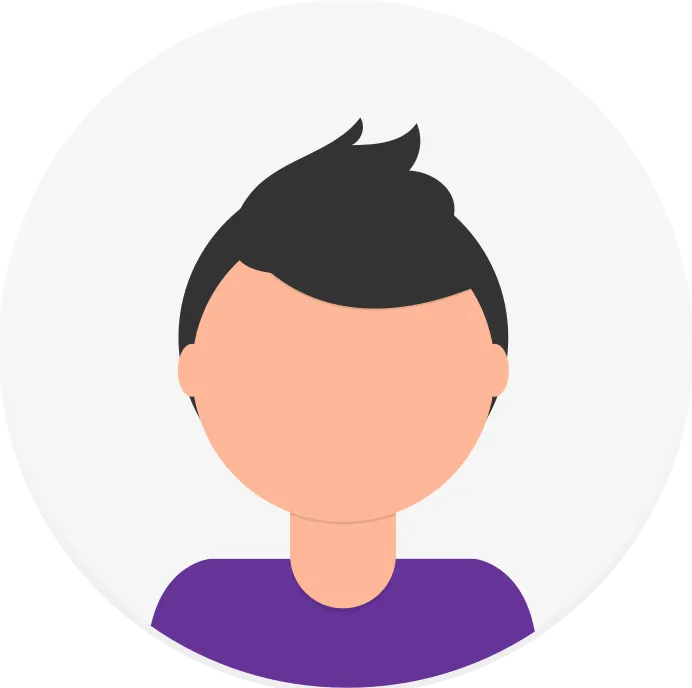
Introduction
Staying up-to-date with the latest technology is crucial with the current advancements in AI. Newer LLM models often introduce cutting-edge features that are only available when using the latest models.
For instance, the ability to generate structured outputs
is only available in more recent versions of OpenAI Models. Attempting to use structured outputs
with outdated models might result in an error:
ERROR : response_format value as json_schema is enabled only for api versions 2024-08-01-preview and later
Legacy Approach: Custom HTTP Handler
In earlier versions of Semantic Kernel (prior to 1.22), the API version was set internally. This meant that updating the package could change the underlying model version being used, potentially breaking existing workflows.
As a workaround for Semantic Kernel lower than version 1.22 developers could implement a custom HTTP handler to set a specific API version. Let’s take a look at how it can be done:
public class AzureOpenAIVersionHandler : DelegatingHandler
{
private const string ApiVersionQueryParam = "api-version";
private readonly string _apiVersionValue;
public AzureOpenAIVersionHandler(string apiVersion) : base(new HttpClientHandler())
{
_apiVersionValue = apiVersion;
}
protected override async Task<HttpResponseMessage> SendAsync(
HttpRequestMessage request,
CancellationToken cancellationToken = default)
{
var uriBuilder = new UriBuilder(request.RequestUri);
var query = HttpUtility.ParseQueryString(uriBuilder.Query);
// Set the api-version to the supplied value
query[ApiVersionQueryParam] = _apiVersionValue;
uriBuilder.Query = query.ToString();
request.RequestUri = uriBuilder.Uri;
return await base.SendAsync(request, cancellationToken);
}
}
This approach ensures that requests to Azure OpenAI use the API version we’ve specified.
To use this custom handler, you would create an instance and pass it to our builder through the AddAzureOpenAIChatCompletion
method:
HttpClient httpClient = new HttpClient(new AzureOpenAIVersionHandler("2024-08-01-preview"));
var builder = Kernel.CreateBuilder();
builder.AddAzureOpenAIChatCompletion(
deploymentName: "gpt-4o",
endpoint: "<ENDPOINT>",
apiKey: "<APIKEY>",
httpClient: httpClient
);
The New Approach: Built-in API Version Support
While we can version control our models by specifying the API version, it wasn’t straightforward. But with recent updates to Semantic Kernel (version 1.22 and later) setting the API version has become much simpler. The AddAzureOpenAIChatCompletion
method now includes a parameter for specifying the API version directly:
var builder = Kernel.CreateBuilder();
builder.AddAzureOpenAIChatCompletion(
deploymentName: "gpt-4o",
endpoint: "<ENDPOINT>",
apiKey: "<APIKEY>",
apiVersion: "2024-08-01-preview"
);
Selecting the appropriate API version is crucial for your use case. Different versions may offer varying features, costs, or performance improvements. To determine which version is most suitable for your needs:
Check the Official Documentation: For the latest Azure OpenAI model updates and version information, refer to the official Microsoft Learn page: https://learn.microsoft.com/en-us/azure/ai-services/openai/concepts/models
Consider Your Requirements: Review the features and capabilities of each version to ensure it meets your project’s needs.
Test Compatibility: Verify that the chosen version is compatible with your current workflow and other dependencies.
Conclusion
Managing API versions effectively is crucial for using the latest features available when using the Azure OpenAI. The new method for setting API versions not only simplifies your code but also ensures that you can quickly adopt new features and improvements as they become available.